Imagine this: you’re on your favorite website, and things change as you move your mouse. Buttons light up, the hidden text shows up, and shapes transform—all because your mouse hovers over them. Isn’t that cool?
This is what we call CSS Hover Effects. It’s not just something you see in movies; you can use it on your websites to make them more interactive.
I will explain how these hover effects work and how you can use them on computers and mobile devices. I’ve also included some real examples and tips to help you make your website look and feel better.
Also Read:
Syntax of the CSS :hover Pseudo-Class
The CSS :hover pseudo-class is straightforward to implement. You simply select the element you wish to apply the effect to, followed by “:hover
“, and then declare the styles you want to apply within curly braces {}. you can see in the given code that I have taken the paragraph (<p>
) tag and targeted it in CSS by class name and applied the :hover effect.
<!DOCTYPE html>
<html>
<head>
<style>
body {background-color: #EAE7DB;}
.hoverEffect:hover {
color: blue;
}
</style>
<body>
<p class="hoverEffect">Deleted text</p>
</body>
</head>
</html>
CSSIn this example, the color of the text will change to blue when the mouse pointer hovers over it.
Examples of Hover Effect
You can manipulate a vast array of properties using the :hover pseudo-class
. Here are a few examples:
Changing Color
One of the simplest and most common hover effects is changing the color of an element. In the following example, we change the text color of a hyperlink:
a:hover {
color: red;
}
CSSIn this case, the text color of the hyperlink will change to red when the mouse hovers over it.
Modifying Font-Size
You can also modify the font-size
of an element when a user hovers over it. This effect can create a visually pleasing emphasis on the hovered element.
<!DOCTYPE html>
<html>
<head>
<style>
body {background-color: #EAE7DB;}
.hoverEffect:hover {
font-size: 18px;}
</style>
<body>
<p class="hoverEffect">Deleted text</p>
</body>
</head>
</html>
CSSIn this example, the font size of a paragraph will increase to 18px when the mouse pointer hovers over it.
Altering Background-Color
Changing the background color of an element upon hover can provide a clear visual cue that an element is interactive. This technique is frequently used in navigation menus and buttons.
button:hover { background-color: lightgray;}
CSSThe background color of a button will change to light gray when it’s hovered over.
Changing Button Color
An essential application of hover effects is changing button colors to signal to the user that the button is interactive. The change can be subtle or distinct, depending on the design requirements. Here’s an example of how you might do this:
<button class="colorButton">Hover Over Me!</button>
HTML.colorButton:hover {
background-color: limegreen;
color: white; }
CSSIn this example, the button color changes to lime green and the text color to white when hovered over, making for a strong visual contrast.
Showing Hidden Information
Another cool trick you can do with CSS hover effects is to reveal hidden information. This is handy for things like image captions, tooltips, or more detailed information about a product in an online store.
<!DOCTYPE html>
<html>
<head>
<style>
body {background-color: #EAE7DB;}
.infoBox .hiddenInfo {
display: none; }
.infoBox:hover .hiddenInfo {
display: block; }
</style>
<body>
<div class="infoBox">
Hover over me
<span class="hiddenInfo">Surprise!</span>
</div>
</body>
</head>
</html>
CSSIn this example, the text “Surprise!” is hidden until you hover over the containing box, at which point it appears.
Output:
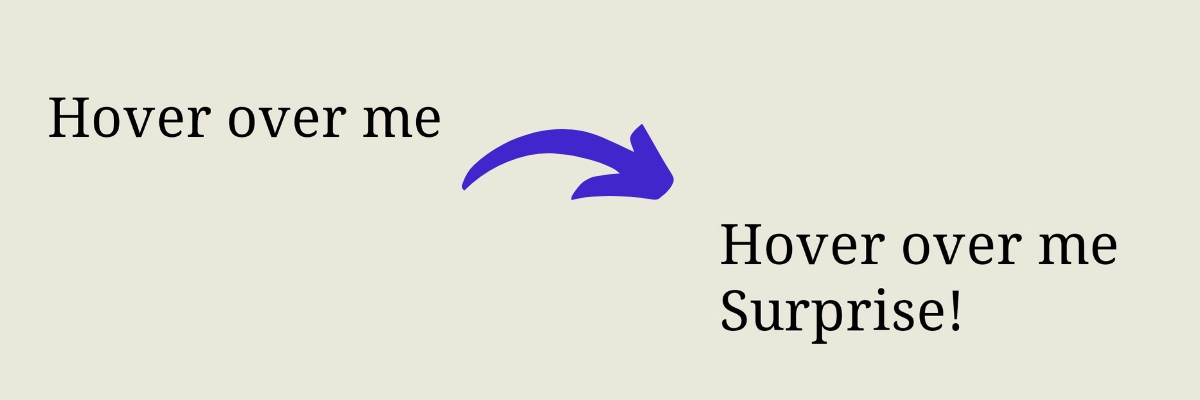
Advanced Button Hover Effects: Transitions and Animations
For more complex hover effects, CSS transitions and animations are your best friends.
Transitions allow you to change property values smoothly, over a specified duration. For instance, if you want a button to slowly change color when you hover over it, rather than changing instantly, you could do something like this:
In this case, the button’s background color will change to yellow over a period of 2 seconds when the mouse hovers over it.
Animations take things a step further. They allow you to gradually change from one style to another, with as many intermediate style changes as you like. Here’s a simple example of a pulsing button.
<!DOCTYPE html>
<html>
<head>
<style>
body {background-color: #EAE7DB;}
button {
transition: background-color 2s;
}
button:hover {
background-color: yellow; }
@keyframes pulse {
0% { transform: scale(1); }
50% { transform: scale(1.1); }
100% { transform: scale(1); }
}
button:hover {
animation: pulse 2s infinite;
}
</style>
<body>
<button>Hover Over Me!</button>
</body>
</head>
</html>
CSSWhen the button is hovered over, it will continuously “pulse” by scaling up and down in size.
How to add Text When Hovering Image css?
We use the css :hover
pseudo-class and the opacity
property to display the text while hovering over the image. Let’s take an example of an image with a caption that appears when hovering over it.
<div class="imageContainer">
<img src="your-image.jpg" alt="Your image">
<div class="caption">This is your image caption</div>
</div>
HTMLIn the above HTML code, I have used an image and caption text wrapped inside a container dev.
/* Define the imageContainer position as relative */
.imageContainer {
position: relative;
display: inline-block;
}
/* Initially, set the caption to be transparent */
.caption {
position: absolute;
bottom: 0;
left: 0;
background: rgba(0, 0, 0, 0.5); /* semi-transparent black background */
color: #fff; /* white text */
width: 100%;
padding: 10px;
text-align: center;
opacity: 0;
transition: opacity 0.5s; /* animate the opacity change */
}
/* Show the caption when hovering over the imageContainer */
.imageContainer:hover .caption {
opacity: 1; /* make the caption fully visible */
}
CSSIn the above CSS code, I initially hide the caption by setting its opacity to 0. When hover over the .imageContainer
, the opacity
of the .caption
changes to 1
, making the caption visible. The transition property is used to animate the opacity change, giving it a smooth fading effect.
Remember to replace "your-image.jpg"
and "Your image"
with your own image file and alt
text.
How can I Change Speed For Hovering in CSS?
The speed of hover effects is controlled by the transition-duration
property. This property specifies how long a transition effect should take to complete.
Here is the code of how to use transition-duration to control the speed of a hover effect:
.button {
background-color: blue;
color: white;
/* Set the transition duration to 2 seconds */
transition: background-color 2s;
}
.button:hover {
background-color: red;
}
CSSIn this example, when we hover over an element with the button
class, its background color will transition from blue to red over a period of 2 seconds.
You can adjust the duration to any value you like, specified in seconds (s) or milliseconds (ms). For example, if you want the transition to take half a second, you would write transition: background-color 0.5s;
.
Remember that the transition
shorthand property includes the transition-property
(the CSS property to transition), and the transition-duration
(the duration of the transition). You can also specify the transition-timing-function
(the speed curve of the transition) and the transition-delay
(the delay before the transition will start). For example:
.button {
background-color: blue;
color: white;
/* Set the transition duration to 2 seconds, with a 1 second delay, using an ease-in-out timing function */
transition: background-color 2s ease-in-out 1s;
}
.button:hover {
background-color: red;
}
CSSIn this updated code, there’s a delay of 1 second before the transition starts, and the speed of the transition follows an “ease-in-out
” timing function, which means the transition starts slowly, speeds up in the middle and then slows down again towards the end.
How to Change the Cursor on Hover in CSS?
You can change the cursor on hover in CSS using the cursor
property. The cursor property specifies the type of cursor to be displayed when pointing over an element.
I have shared a simple example of changing the cursor to a pointer (hand cursor) when hovering over a button.
.button {
background-color: blue;
color: white;
/* Set the transition duration to 2 seconds, with a 1 second delay, using an ease-in-out timing function */
transition: background-color 2s ease-in-out 1s;
}
.button:hover {
background-color: red;
}
CSSIn this case, when hovering over an element with the button
class, the cursor will change to a pointer.
There are many different cursor styles you can use, such as:
default
: The default cursor (usually an arrow)pointer
: A pointer (hand cursor, often used for links)wait
: A wait cursor (usually a watch or hourglass)text
: A text cursor (usually an I-beam, used over text)move
: A move cursor (usually a cross with arrows, used for movable items)not-allowed
: A “not allowed” cursor (usually a circle with a line through it)
Here is the code for different styles of cursors. You can choose as you like:
.defaultCursor:hover {
cursor: default;
}
.pointerCursor:hover {
cursor: pointer;
}
.waitCursor:hover {
cursor: wait;
}
.textCursor:hover {
cursor: text;
}
.moveCursor:hover {
cursor: move;
}
.notAllowedCursor:hover {
cursor: not-allowed;
}
CSSJust apply the appropriate class to the HTML elements you want to have these hover effects. When you hover over these elements, the cursor will change to the specified style.
For example, the following button will show a not-allowed
cursor when you hover over it:
<button class="notAllowedCursor">Can't Click Me!</button>
HTMLI hope this guide has easily helped you in making your hover effects. If you face any problems or have any questions, don’t hesitate to discuss them in the comments!