Are you ready to brush up on your HTML and CSS skills? Here I am again with an immersive tutorial, I am going to create a responsive checkout form from scratch. This is not just an exercise in form building; This is a practical lesson in developing a critical component of any e-commerce platform – a component that performs flawlessly on any device. From the basics of form layout and validation to mastering CSS styling and responsiveness, I’ve got it all covered.
I am 100% confident that by the end of this tutorial, you will be well equipped to create your own responsive checkout forms, ensuring a smooth, user-friendly experience regardless of screen size. So, roll up your sleeves and let’s dive into the exciting world of responsive web design. 😆
How to create Checkout Form Structure
When I began crafting the HTML structure, my first step was to establish a foundational div
element with the class name container
. This div
serves as the primary container for the entire form. It’s like the canvas on which all the other elements of our form will be painted. This container is crucial because it provides a centralized and organized space where all the other elements of our form can reside.
Also read: Examples of CSS Contact Forms
Order Info
Now I moved on to the first step of our form. I added a div
element with the class order-info. This div is essentially a container for the order summary. Inside this div, I placed 4 more tags. I used an h2
tag for the title “Order Summary”, an img tag to display an image of the product, and p
tags to display the product name and details and also added an h3
tag to show the product price and closed the order-info div
tag.
<div class="container">
<div class="order-info">
<h2>Order Summary</h2>
<img src="https://dl.dropboxusercontent.com/s/sim84r2xfedj99n/%24_32.JPG" class="full-width">
<p>Nike Free Run 3.0 Women</p>
<p>Color: Grey/Orange, Size: 10.5</p>
<h3 class="price">$500</h3>
</div>
HTMLIn CSS part, I styled the .container class. I used flexbox to align the elements, You can use grid layout instead of flex but i like flex so i used it. Set the width to 80% of its parent’s width but not more than 800px, added a subtle shadow around the container, set the background color to white, and added some padding.
.container {
display: flex;
justify-content: space-between;
width: 80%;
max-width: 800px;
box-shadow: 0 0 10px rgba(0,0,0,0.1);
background-color: #fff;
padding: 20px;
}
CSSStyling the order info Image
I set the width of the image to 100% of its parent’s width, which makes it fill the entire width of the order info section.
.order-info img {
width: 100%;
}
CSSOutput:
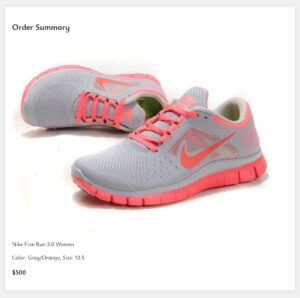
How to create Checkout Form in Html?
Next, I created another div
with the class checkout-form
. This div
is a container for the checkout form. Inside this div, I used a form tag to create an HTML form for user input. Then I added 4 input tags to create fields where users can enter their card number, cardholder name, expiry date, and CVV.
As you can see I have added some attributes (type, id, placeholder, required
) in each input tags.
- The
type
attribute specifies the type of input to display. - The
id
attribute assigns an ID to the input (which can be used in JavaScript and CSS). Placeholder
attribute provides a short hint that describes the expected value of the input field.- The “
required
” attribute ensure users provide necessary information in an input field. If a user attempts to submit the form by clicking the “Pay” button without entering any information in a field marked as “required”, the browser will intervene. A pop-up message will appear, prompting the user to “please fill out this field”, thereby preventing the form from being submitted until all required fields are filled.
<div class="checkout-form">
<h2>Checkout</h2>
<form>
<input type="text" id="card-number" placeholder="Card Number" required>
<input type="text" id="card-name" placeholder="Cardholder Name" required>
<input type="text" id="expiry-date" placeholder="Expiry Date" required>
<input type="text" id="cvv" placeholder="CVV" required>
...
</form>
</div>
HTMLStyling the Order Info and Checkout Form
Let’s turn to styling the .order-info
and .checkout-form
classes, which are the two main sections of our content.
I set the width: 50%;
of each section to 50% of the container’s width, which makes them each take up half of the container and added some other basic design you can follow the codes.
.order-info, .checkout-form {
width: 50%;
padding: 10px;
}
.checkout-form {
background-color: #614CCD;
}
.checkout-form h2 {
color: white;
}
CSSOutput:

How to Style the Form Inputs?
I added margin and padding to increase the space around them, removed the default borders, and added rounded corners for a modern touch. Also, I added a subtle shadow for a 3D effect and increased the font size for readability. To ensure the fields filled their container, I set their width to 100% and used box-sizing: border-box to include padding and border in the total width and height. This approach gives us a sleek, modern form, but remember that the customization options are endless. You can adjust these styles to create a unique look that fits your needs.
.checkout-form input {
margin-bottom: 15px;
padding: 10px;
border: none;
border-radius: 5px;
box-shadow: 0 0 15px rgba(0,0,0,0.1);
font-size: 16px;
width: 100%;
box-sizing: border-box;
}
CSSHow to create a submit button in HTML?
Creating a submit button in HTML is straightforward. You can use the <button>
element with the attribute type="submit"
within a <form>
element.
Finally I added button
tag. The <button> tag is used to create a clickable button. The type=”submit” attribute specifies that it’s a submit button, which will submit the form data when clicked.
<button type="submit">Pay</button>
HTMLStyling Button
I started by increasing the padding to make it larger and easier to click. I removed the default border and added rounded corners for a modern look. I chose a shade of Pearl Aqua for the background color and set the text color to white for contrast. I increased the font size for readability and changed the cursor to a pointer to indicate clickability. I also added a transition to the background color for a smooth hover effect. Finally, I used the :hover
pseudo-class to change the background color to a darker shade of blue when the button is hovered over. This combination of CSS properties – padding, border, border-radius, background-color, color, font-size, cursor, transition, and hover – gives the button a sleek, modern look.
.checkout-form button {
padding: 12px 20px;
border: none;
border-radius: 5px;
background-color: #007BFF;
color: #fff;
font-size: 16px;
cursor: pointer;
transition: background-color 0.3s ease;
}
.checkout-form button:hover {
background-color: #0056b3;
}
CSSCard Type Selection
When I decided to add a selection for the card type, I thought about the most common payment methods people use. I chose American Express, Visa Card, and PayPal. I created a new div
with the class card-selection
to contain the selection. Inside this div
, I added a p
tag to prompt the user to select a card type. Then, I used a select
tag to create a dropdown list for the card types. I used option
tags to define the available options in the dropdown list.
<div class="card-selection">
<p>Select Card Type:</p>
<select id="card-type">
<option value="american-express">American Express</option>
<option value="visa">Visa Card</option>
<option value="paypal">PayPal</option>
</select>
</div>
HTMLFor the dropdown itself, I added some padding around the text. I removed the default border and rounded the corners with border-radius
. To give the dropdown a 3D effect, I added a subtle shadow around it with box-shadow. I increased the size of the text inside the dropdown with font-size and made the dropdown take up the full width of its container with width.
.card-selection {
display: flex;
align-items: center;
margin-bottom: 15px;
}
.card-selection p {
margin-right: 10px;
}
.card-selection select {
padding: 10px;
border: none;
border-radius: 5px;
box-shadow: 0 0 15px rgba(0,0,0,0.1);
font-size: 16px;
width: 100%;
box-sizing: border-box;
appearance: none;
background: url('dropdown-arrow.png') no-repeat right;
background-size: 12px;
}
CSSOutput:
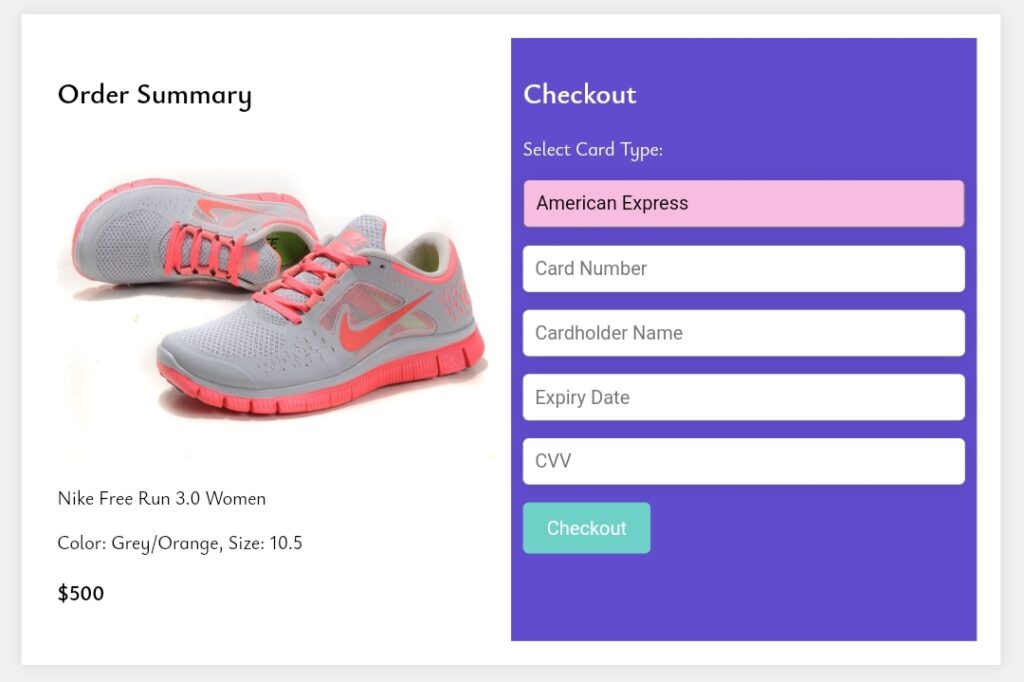
How to Create a Responsive Checkout Form?
Now, I’ve arrived at the final step. At this point, the form isn’t fully optimized for all devices, so my next task is to make it responsive. To achieve this, I’m going to use CSS media queries. This powerful tool will allow me to ensure the form adapts perfectly to any screen size, providing an optimal user experience across all devices.
This media query I’m using makes sure that when the screen size is 600 pixels or less – a common scenario for mobile devices in portrait mode – the layout of the form adapts. The order summary and checkout form, which are displayed next to each other on larger screens, will stack vertically for easier navigation on smaller screens. This ensures the form remains user-friendly, no matter the device it’s viewed on.
Also read: Examples of CSS Checkout Form
@media (max-width: 600px) {
.container {
flex-direction: column;
}
.order-info, .checkout-form {
width: 100%;
}
}
CSSTip: Always remember to place your media queries at the end of your CSS.