In this post, I will share with you how to implement the custom design on the checkbox using pure css. Most people think that custom designs do not apply to checkboxes without the use of 3rd party plugins but this thinking is wrong. We can achieve a lot of different custom designs applied to checkboxes using CSS. In this case, you can see how the background and border of the checkbox are designed with the help of css.
What is Checkbox in HTML?
A checkbox in HTML is a form element that is visually represented as a small square box, which can be clicked to select or deselect an option. A checkbox is defined in HTML using the <input type="checkbox">
tag. While often used within forms to capture user choices such as accepting terms and conditions, it is not strictly necessary for checkboxes to be contained within a form tag. it’s actually possible to use them outside of the form tag as well. They won’t submit data if they’re outside a form, but they can still be functional for other purposes, such as toggling visibility of elements through JavaScript.
<input type="checkbox">
What is CSS for Checkbox?
As I above mentioned, a checkbox is an HTML element, and it’s natural to wonder how we can style it and target it in CSS. Trust me, it’s not as difficult as it might seem, although it can be a bit tricky. We can target checkbox in CSS using pseudo-elements. You can also target a specific checkbox through it’s own Id or class name. Join me and read through this entire article. I assure you, by the end of it, you’ll feel confident about designing your own checkboxes.
<input type="checkbox" id="myCheckbox">
CSS:
/* Target through direct Tag. */
input[type="checkbox"] {
/* CSS properties go here */
}
/* Target through specific id name. */
#myCheckbox {
/* CSS pro
In the above code section, I’ve shared two examples of how to target checkboxes in CSS. If you want to target all checkboxes on your website, use a pseudo-element, and want to target a specific checkbox, use its id or class name.
However, the default style of checkboxes can be quite plain and might not align with the visual design of your website. This is where CSS comes in. With CSS, you can transform the humble checkbox into a visually appealing and interactive element that enhances user engagement.
For example, you can use CSS to change the color of the checkbox, adjust its size, modify its shape, or add a hover effect. You can even create a completely custom checkbox design from scratch, all with pure CSS.
Here is the default Checkbox style:

How to Change the Background of a Checkbox in CSS?
In the HTML code, I have a label element with a class of custom-checkbox. Inside this label, I have taken an input element of type checkbox and a span element with a class of checkmark. I’m using a label element so that when the user click on the custom checkbox, it will toggle the input
checkbox.
<label class="custom-checkbox">
<input type="checkbox">
<span class="checkmark"></span>
</label>
Now our tricky part begins. In the first step, we need to hide the default checkbox because we can’t change the style of the default checkbox very much. So, I hide it and create our custom checkbox that we can style however we want. In this code, I’m using position: absolute to remove the checkbox from the normal flow of the document so it doesn’t take up any space. I’m also setting opacity: 0
, height: 0
, and width: 0
to make the checkbox invisible and take up no space.
/* Hide the default checkbox */
.custom-checkbox input {
position: absolute;
opacity: 0;
height: 0;
width: 0;
}
I’m creating a custom checkbox. Here is the core of our custom checkbox style. You can use any type of CSS property in this area that suits your website design. Now I move on to our questions how to change the background color of the checkbox and how to style a custom checkbox using pure css?: I’m using the span element with the class checkmark to do this. I’m setting position: relative so that we can position the checkmark (which we’ll create later) relative to this custom checkbox. I’m adding display: inline-block
so that we can give the span
element a width and height. And finally, I’m using the background-color property to change its bg color and also add the Border property to add a border.
/* Create a custom checkbox */
.custom-checkbox .checkmark {
position: relative;
display: inline-block;
left: 0;
height: 25px;
width: 25px;
background-color: #191D1C;
border: 2px solid #4CAF50;
}
Output:
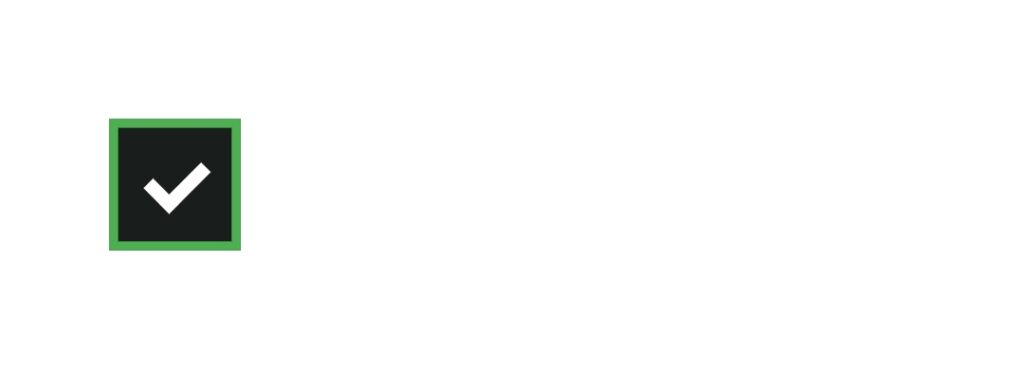
In this part, I’m creating the checkmark that will appear when the checkbox is checked. I’m using the ::after pseudo-element to do this. The content: “” property is necessary for the pseudo-element to work. I’m setting position: absolute to position the checkmark relative to the custom checkbox. I’m initially setting display: none to hide the checkmark. I’m also setting the left, top, width, and height to position and size the checkmark. The border and border-width properties are used to create the checkmark, and the transform: rotate(45deg)
property is used to rotate the checkmark.
/* Create the checkmark */
.custom-checkbox .checkmark:after {
content: "";
position: absolute;
display: none;
left: 9px;
top: 5px;
width: 5px;
height: 10px;
border: solid white;
border-width: 0 3px 3px 0;
transform: rotate(45deg);
}
/* Show the checkmark when the checkbox is checked */
.custom-checkbox input:checked ~ .checkmark:after {
display: block;
}
How to Change the background color when the checkbox is checked?
If you want to change the background color when the checkbox is checked, you can do this:
/* Change the background color when the checkbox is checked */
.custom-checkbox input:checked ~ .checkmark {
background-color: #4CAF50;
border: solid black;
}
In this example, I’m adding the background-color property to set the color of (#4CAF50), which is a shade of green. This means that when the checkbox is checked, the background color of the custom checkbox will change to this color. You can change this to any color you want.
Output:

How to change check color in checkbox CSS?
To change the color of the checkmark in the custom checkbox, you can adjust the border
property in the .checkmark:after
rule. This property is used to create the checkmark, so changing the color here will change the color of the checkmark.
Here’s how you can change the color of the checkmark:
/* Create the checkmark */
.custom-checkbox .checkmark:after {
content: "";
position: absolute;
display: none;
left: 9px;
top: 5px;
width: 5px;
height: 10px;
border: solid #ff0000; /* This is a red color */
border-width: 0 3px 3px 0;
transform: rotate(45deg);
}
How to Change the Checkbox Background color, when checked Without Using Custom CSS?
Absolutely, let’s put it in that way:
If you’re looking to change the color of your checkbox when it’s selected, but don’t want to use custom CSS, there’s a handy CSS property just for you: ‘accent-color
‘. This nifty property allows you to set the background color of your checkbox with just a single line of code.
Just remember, accent-color
is a new CSS property and may not work in all browsers.
input[type="checkbox"] {
accent-color: darkblue;
}
Output:
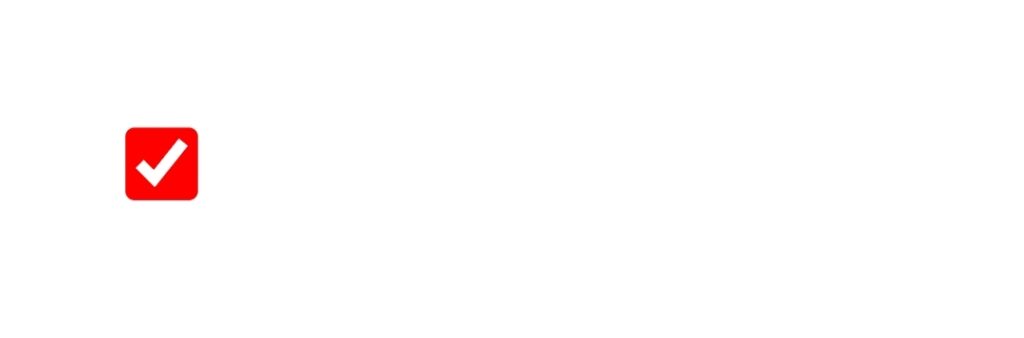